Friday, January 16, 2015
Login using Google Javascript API Sample Code
Latest Google Javascript API allow users to fetch their google profile information, circle details. Javscript API is not just limited to this, but it allow us to fetch and upload Google Drive file. Read more
In this tutorial we are utilizing Google+ Javascript API to fetch user Google+ Profile Details and Email address. It is purely OAuth2 Compatible login method and its secure.
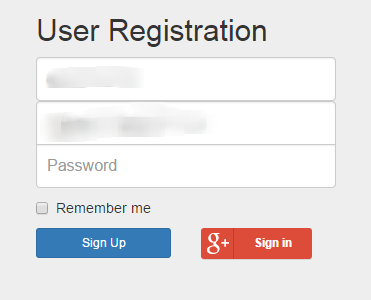
Before you begin this tutorial, you actually need to setup a Project through Google Developer Console. You can follow google docs here.
Step 1: Create Project
Now Client Key and Secret key successfully created.
Note: For javascript based login, we dont use Secret key, only Client Key is enough.
Javascript Code for the Google+ API and Fetching User Details, Email from Google Profile
If you click on the Google+ Login Button it will show a popup like below. This means your configuration in google developer console is right.
Thats it.
In this tutorial we are utilizing Google+ Javascript API to fetch user Google+ Profile Details and Email address. It is purely OAuth2 Compatible login method and its secure.
Workflow is simple
- User Clicks on Login with Google Button on XYZ Website
- Google OAuth2 Popup opens and ask the user login to google account first.
- If Logged in, it shows the permissions that this website is requesting, then user accept this by pressing button Allow.
- Now the website receives the user information which are requested by the XYZ Website ( Login Scope )
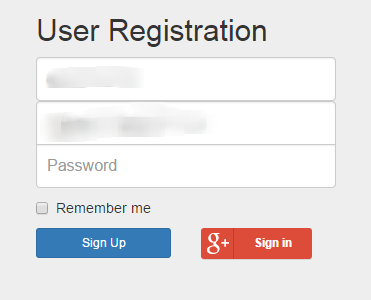
Before you begin this tutorial, you actually need to setup a Project through Google Developer Console. You can follow google docs here.
Step 1: Create Project
Step 2: Set the Consent Screen details
Step 3: Create Web application Client Key and Secret
Now Client Key and Secret key successfully created.
Note: For javascript based login, we dont use Secret key, only Client Key is enough.
How to Google+ Javascript Login
Add Google+ Javascript API Javascript Header
<script type="text/javascript">
(function() {
var po = document.createElement(script);
po.type = text/javascript; po.async = true;
po.src = https://plus.google.com/js/client:plusone.js;
var s = document.getElementsByTagName(script)[0];
s.parentNode.insertBefore(po, s);
})();
</script>
Add JQuery & Bootstrap for styling
<!-- Bootstrap core CSS -->
<link href="http://getbootstrap.com/dist/css/bootstrap.min.css" rel="stylesheet">
<!-- Custom styles for this template -->
<link href="http://getbootstrap.com/examples/signin/signin.css" rel="stylesheet">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="http://getbootstrap.com/dist/js/bootstrap.min.js"></script>
Complete HTML Body code for the Form and Google+ Button
<div class="container">
<form class="form-signin" role="form">
<div id="status"></div>
<h2 class="form-signin-heading">User Registration</h2>
<label for="inputFname" class="sr-only">First Name</label>
<input type="text" id="inputFullname" class="form-control" placeholder="First Name" required autofocus>
<label for="inputEmail" class="sr-only">Email address</label>
<input type="email" id="inputEmail" class="form-control" placeholder="Email address" required >
<label for="inputPassword" class="sr-only">Password</label>
<input type="password" id="inputPassword" class="form-control" placeholder="Password" required>
<div class="row">
<div class="col-md-6">
<button class="btn btn-sm btn-primary btn-block" type="submit">Sign Up</button>
</div>
<div class="col-md-6">
<button class="g-signin "
data-scope="https://www.googleapis.com/auth/plus.login https://www.googleapis.com/auth/userinfo.email"
data-requestvisibleactions="http://schemas.google.com/AddActivity"
data-clientId="1049178870057-usbfluijl3qtq3nijmucnsksr9gvkag4.apps.googleusercontent.com"
data-accesstype="offline"
data-callback="mycoddeSignIn"
data-theme="dark"
data-cookiepolicy="single_host_origin">
</button>
</div>
</div>
</form>
</div> <!-- /container -->
Javascript Code for the Google+ API and Fetching User Details, Email from Google Profile
<script type="text/javascript">
var gpclass = (function(){
//Defining Class Variables here
var response = undefined;
return {
//Class functions / Objects
mycoddeSignIn:function(response){
// The user is signed in
if (response[access_token]) {
//Get User Info from Google Plus API
gapi.client.load(plus,v1,this.getUserInformation);
} else if (response[error]) {
// There was an error, which means the user is not signed in.
//alert(There was an error: + authResult[error]);
}
},
getUserInformation: function(){
var request = gapi.client.plus.people.get( {userId : me} );
request.execute( function(profile) {
var email = profile[emails].filter(function(v) {
return v.type === account; // Filter out the primary email
})[0].value;
var fName = profile.displayName;
$("#inputFullname").val(fName);
$("#inputEmail").val(email);
});
}
}; //End of Return
})();
function mycoddeSignIn(gpSignInResponse){
gpclass.mycoddeSignIn(gpSignInResponse);
}
</script>
If you click on the Google+ Login Button it will show a popup like below. This means your configuration in google developer console is right.
![]() |
Login using Google Popup |
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.